Appreciating Rails test scaffolds
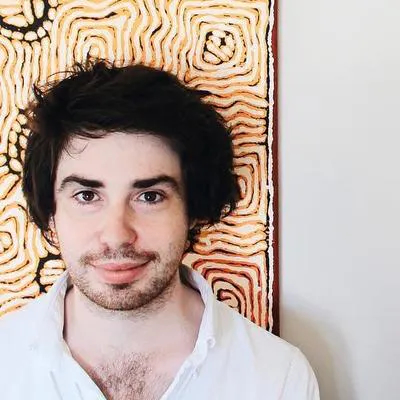
Dwight Watson
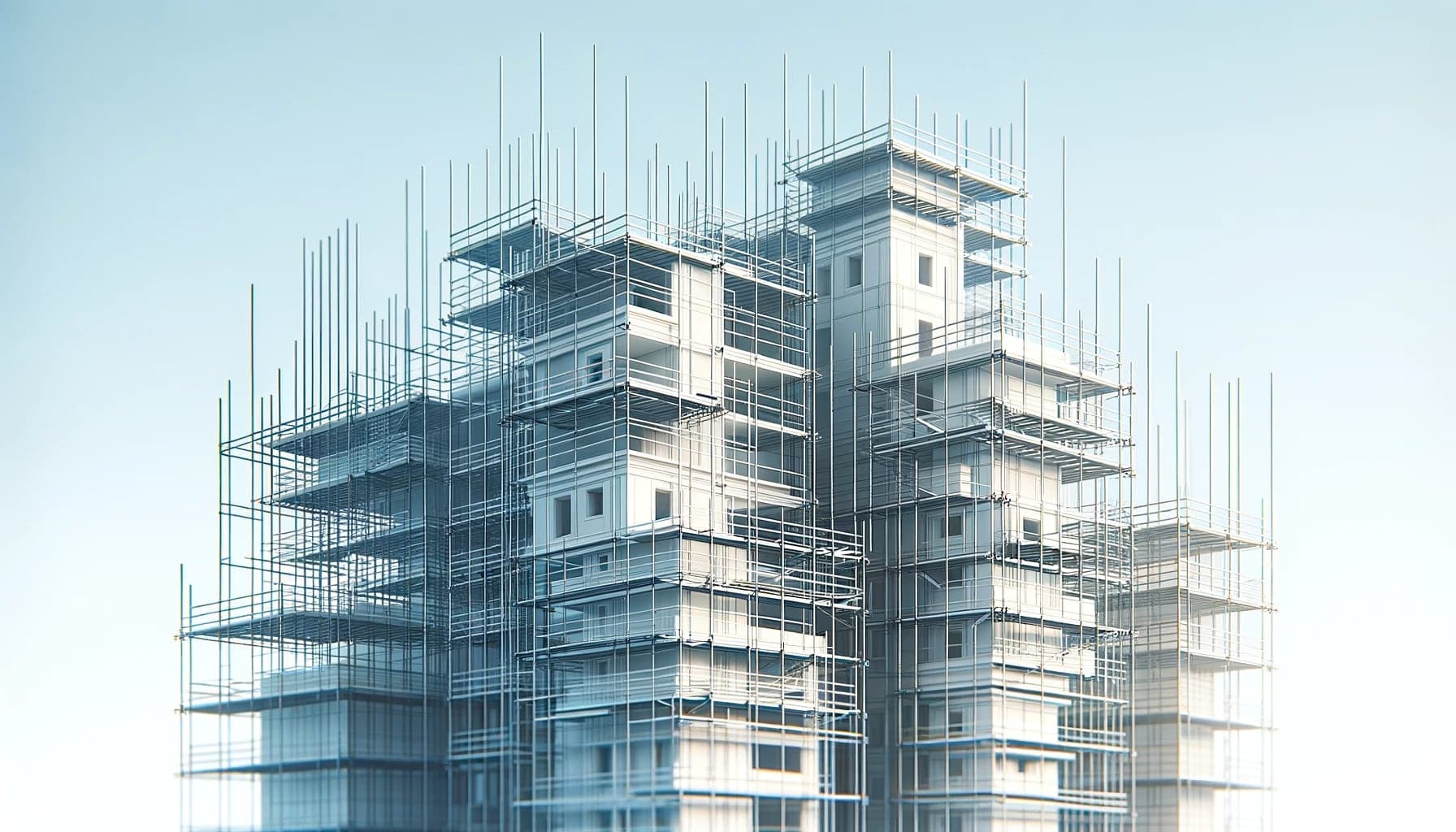
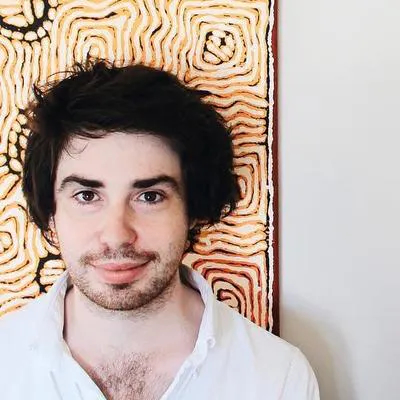
Dwight Watson
When using the generate scaffold
command from Rails it will also generate a controller and a system test. It's worth paying attention to these instead of just discarding them. They are a useful learning tool for a few reasons.
- They immediately provide free test coverage for the happy path
- They help guide towards Rails conventions - simple controller tests that test the entire request
- They help teach you how to use Minitest
Controller test
require "test_helper"
class PostsControllerTest < ActionDispatch::IntegrationTest
setup do
@post = posts(:one)
end
test "should get index" do
get posts_url
assert_response :success
end
test "should get new" do
get new_post_url
assert_response :success
end
test "should create post" do
assert_difference("Post.count") do
post posts_url, params: { post: { title: @post.title } }
end
assert_redirected_to post_url(Post.last)
end
test "should show post" do
get post_url(@post)
assert_response :success
end
test "should get edit" do
get edit_post_url(@post)
assert_response :success
end
test "should update post" do
patch post_url(@post), params: { post: { title: @post.title } }
assert_redirected_to post_url(@post)
end
test "should destroy post" do
assert_difference("Post.count", -1) do
delete post_url(@post)
end
assert_redirected_to posts_url
end
end
System test
require "application_system_test_case"
class PostsTest < ApplicationSystemTestCase
setup do
@post = posts(:one)
end
test "visiting the index" do
visit posts_url
assert_selector "h1", text: "Posts"
end
test "should create post" do
visit posts_url
click_on "New post"
fill_in "Title", with: @post.title
click_on "Create Post"
assert_text "Post was successfully created"
click_on "Back"
end
test "should update Post" do
visit post_url(@post)
click_on "Edit this post", match: :first
fill_in "Title", with: @post.title
click_on "Update Post"
assert_text "Post was successfully updated"
click_on "Back"
end
test "should destroy Post" do
visit post_url(@post)
click_on "Destroy this post", match: :first
assert_text "Post was successfully destroyed"
end
end